Node Web Server - Localhost HTTPS
Created: January 10, 2020
Updated: April 16, 2020
To better protect end-users, we have to make sure that communication between a browser and our server is encrypted — this is where https comes in. HTTPS requires SSL certificates issued by Certificate Authority which LetsEncrypt makes quite easy to install on a production environment.
A node application usually sits behind a proxy, so https may not be needed. However, as developers, we sometimes have to support https on our local environment for development and debugging purposes.
Before we start coding, we first need to create a self-signed certificate.
Fortunately, mkcert
makes this possible and easier than remembering openssl commands.
Of course, it's important to know how certificates are created, but we're taking the easy route.
Let's install mkcert
.
If you don't have a node development environment set-up, you should read Node Development Environment Set-up - For Windows.
Run a powershell terminal as an administrator, and type the following command:
>choco install mkcert
Install our root certificate.
>mkcert -install
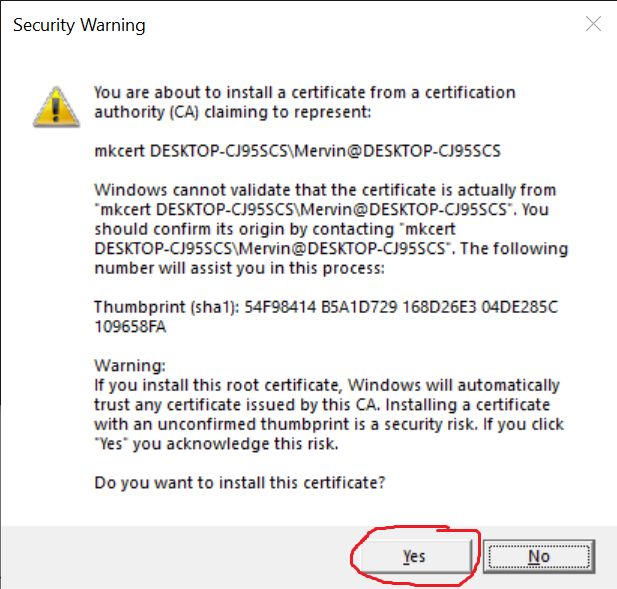
A security warning will pop up. Click Yes to continue installation.
Just like that, we have a root certificate that will sign all the certificates that we are going to create locally. To check the installation directory, type the following command:
>mkcert -CAROOT
C:\Users\Mervin\AppData\Local\mkcert
On my computer, the root certificate was installed in the C:\Users\Mervin\AppData\Local\mkcert
folder.
Let's change to that directory, and create a certificate for localhost. We can put our certificates anywhere,
we will keep them with the root certificate.
>cd ~/AppData\Local\mkcert>mkcert localhost
The command above created two files: localhost-key.pem
and localhost.pem
.
We will use these files to give credentials to our https server.
Now, we are ready to create our https project. Run a powershell terminal, and change to your home directory
>cd ~/
Create our project folder, and change to that directory
>mkdir https-server>cd https-server
Initialize our project with default package.json
>npm init -y
Open the current folder in visual studio code.
>code .
Open package.json. Change the value of main
field from index.js
to server.js
.
Let's also add a field type
, and set its value to module
so we can code in es6 format.
The package.json file should look like the following:
1{2 "name": "https-server",3 "version": "1.0.0",4 "description": "",5 "main": "server.js",6 "type": "module",7 "scripts": {8 "test": "echo \"Error: no test specified\" && exit 1"9 },10 "keywords": [],11 "author": "",12 "license": "ISC"13}
Let's create server.js
in the root directory of the project then copy and paste the following code.
Don't forget to change my username to your username for paths to localhost-key.pem
and localhost.pem
.
1// server.js2import https from 'https'3import fs from 'fs';45const privateKey = fs.readFileSync('C://Users/Mervin/AppData/Local/mkcert/localhost-key.pem', 'utf8');6const certificate = fs.readFileSync('C://Users/Mervin/AppData/Local/mkcert/localhost.pem', 'utf8');7const credentials = {key: privateKey, cert: certificate};89const httpsServer = https.createServer(credentials, (req, res) => {10 res.statusCode = 200; // OK11 res.setHeader('Content-Type', 'text/plain');12 res.write("Hello world!");13 res.end();14});1516httpsServer.listen(4001, () => {17 console.debug("HTTP Server running on port 4001.");18})
On the menubar, click Run -> Start without Debugging or press Ctrl + F5 to run the program.
Now, go back to the browser and type https://localhost:4001 on the adress bar and press enter. If you're using Edge or Chrome, you should see "Hello world!" displayed on the screen. With firefox, we still need to add our root certificate to the list of authorities; hence, this warning.
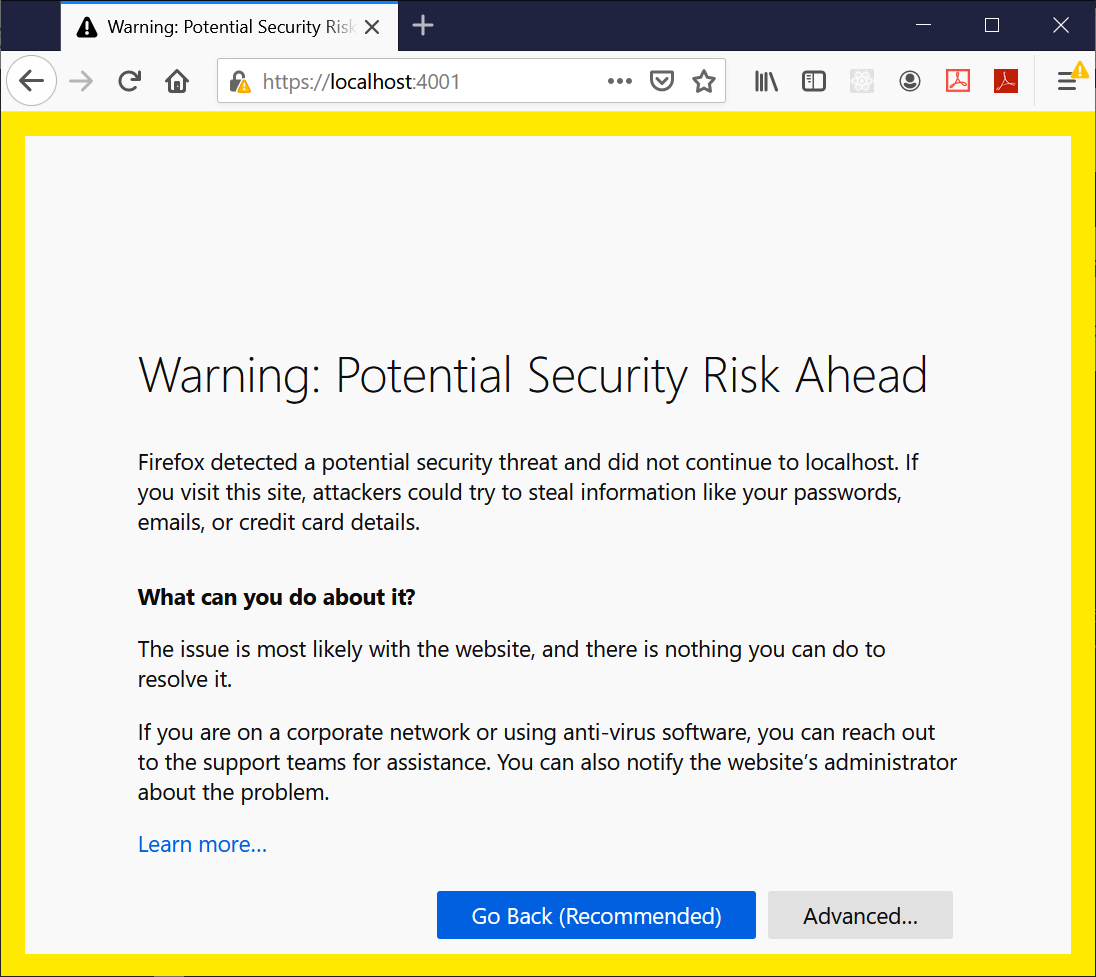
To fix the firefox warning, do the following:
- Open a firefox browser and type about:preferences#privacy on the address bar.
- Scroll to bottom and click on View Certificates.
- Select the Authorities tab.
- Click Import.
- Browse to where we installed our root certificate. In my case, it's
C:\Users\Mervin\AppData\Local\mkcert
- Select rootCA.pem
- Check Trust this CA to identify websites.
- Click OK
That's it pancit! Just like that, we created an https server on localhost.
Previous